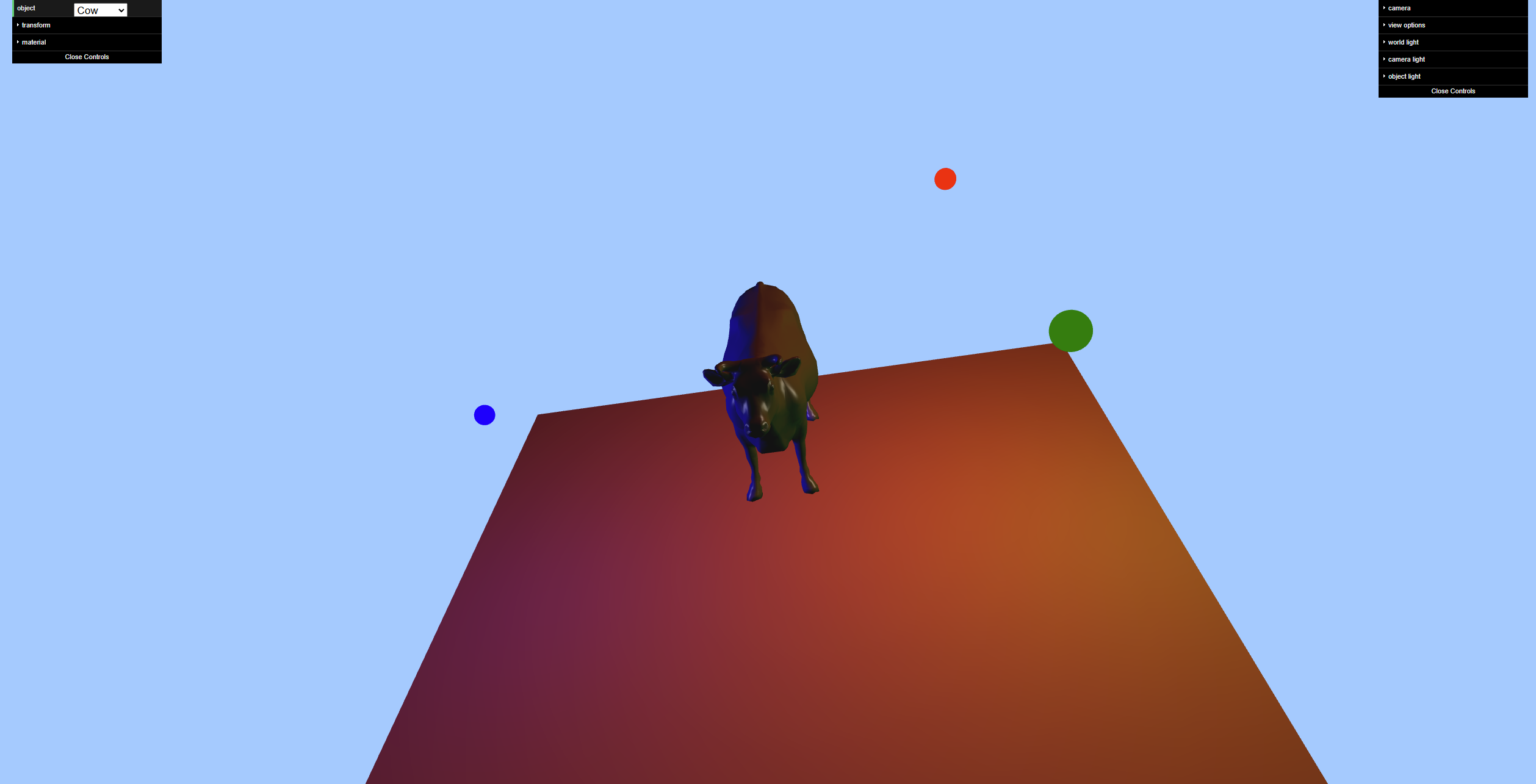
Check it out -> Phong and Gouraud Shading
Phong and Gouraud Shading in WebGL
Introduction
This project implements Phong and Gouraud shading in WebGL, allowing real-time lighting calculations using the Phong illumination model. The user can switch between:
- Gouraud Shading – Lighting is calculated per vertex and interpolated across fragments.
- Phong Shading – Lighting is calculated per fragment for more accurate shading.
The application also allows scene modifications, including geometric transformations, light configuration, and camera control.
Features
-
Object Manipulation
- Apply translation, rotation, and scaling.
- Adjust material properties (
Ka
,Kd
,Ks
,shininess
) used in the Phong illumination model.
-
Configurable Lighting
- Three independently controlled light sources:
- World space light
- Object space light (transforms with the object)
- Camera space light (transforms with the camera)
- Toggle between point and directional lights.
- Adjust light intensity, position, and orientation.
- Three independently controlled light sources:
-
Visualization Options
- Enable or disable:
- Back-face culling
- Z-buffer
- Light source visualization (small spheres representing light positions).
- Enable or disable:
-
Camera Control
- Rotation: Left mouse button
- Zoom: Mouse scroll
- Adjust perspective projection parameters (fovy, near, far) via the interface.
Implementation
The project uses WebGL and dat.gui for the graphical interface. The shaders support both shading techniques:
Implemented Shaders
📌 phong.vert
- Vertex Shader
- Transforms vertex positions and normal vectors into camera space.
- Sends necessary data to the Fragment Shader.
📌 phong.frag
- Fragment Shader
- Computes Phong lighting per fragment for high-quality shading.
📌 gouraud.vert
- Vertex Shader
- Computes lighting per vertex and interpolates the result across the surface.
📌 gouraud.frag
- Fragment Shader
- Simply applies the interpolated vertex colors.
📌 lightShader.vert
& lightShader.frag
- Handles visualization of light positions in the scene.